Element Sizes & Scrolling
Sample element
<div id="example">...Text...</div>
<style>
#example {
width: 300px;
height: 200px;
border: 25px solid #e6e6e6;
padding: 20px;
overflow: auto;
}
</style>
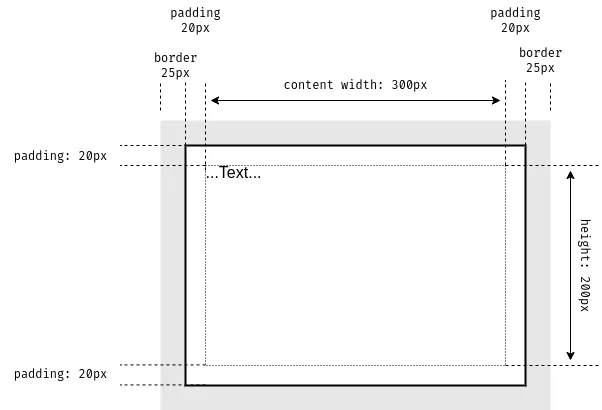
Mind the scrollbar. If the scrollbar is
16px
wide then the content width remains only300 - 16 = 284px
.
Geometry
Here's the overall picture with geometry properties:
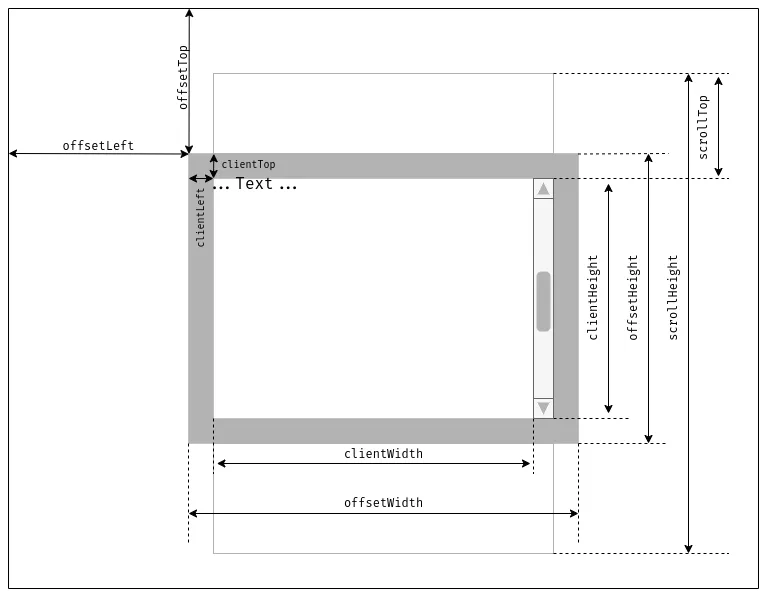
offsetWidth
: The outer width = inner CSS-width + paddings + bordersoffsetHeight
: The outer height = inner CSS-height + paddings + bordersclientLeft
: Left border widthclientTop
: Top border widthclientWidth
: The inner width = inner CSS-width + paddings (exclude scrollbar)clientHeight
: The inner height = inner CSS-height + paddings (exclude scrollbar)scrollWidth
: The full inner width including the scrolled out partsscrollHeight
: The full inner height including the scrolled out partsscrollTop
: How much is scrolled upscrollLeft
: How much is scrolled left
Don't take width/height from CSS
We should use geometry properties instead of getComputedStyle
:
CSS
width/height
depend on another property:box-sizing
that defineswhat is
CSS width andCSS
width/height
maybeauto
A scrollbar
Window sizes and scrolling
Width/height of the window
documentElement.clientHeight;
documentElement.clientWidth;
Not
window.innerWidth/innerHeight
alert(window.innerWidth); // full window width alert(document.documentElement.clientWidth); // window width minus the scrollbar
Width/height of the document
Theoretically, as the root document element is document.documentElement
, and it encloses all the content, we could measure the document's full size as document.documentElement.scrollWidth/scrollHeight
.
But on that element, for the whole page, these properties do not work as intended. In Chrome/Safari/Opera, if there's no scroll, then documentElement.scrollHeight
may be even less than documentElement.clientHeight
! Weird, right?
To reliably obtain the full document height. we should take the maximum of these properties:
let scrollHeight = Math.max(
document.body.scrollHeight,
document.documentElement.scrollHeight,
document.body.offsetHeight,
document.documentElement.offsetHeight,
document.body.clientHeight,
document.documentElement.clientHeight
);
Get the current scroll
DOM elements have their current scroll state in their scrollLeft/scrollTop
properties.
For document scroll: document.documentElement.scrollLeft/scrollTop
Speical properties: window.pageXOffset/pageYOffset
For historical reasons, these properties are the same:
window.pageXOffset
is an alias ofwindow.scrollX
window.pageYOffset
is an alias ofwindow.scrollY
Scrolling: scrollTo, scrollBy, scrollIntoView
window.scrollBy(x,y)
- scrolls the page relative to its current positionwidnow.scrollTo(pageX, pageY)
- scrolls the page to absolute coordinates, so that the top-left corner of the visible part has coordinates(pageX, pageY)
ralative to the document's top-left corner. It's like settingscrollLeft/scrollTop
scrollIntoView
The call to elem.scrollIntoView(top)
scrolls the page to make elem
visible. It has one argument:
If
top=true
(default), then the page will be scrolled to makeelem
appear on the top of the window. The upper edge of the element will be aligned with the window top.If
top=false
, then the page scrolls to makeelem
appear at the bottom. The bottom edge of the element will be aligned with the window bottom.
Forbid the scrolling
document.body.style.overflow = "hidden";