Node Properties
DOM node classes
Different DOM nodes may have different properties. For instance, an element node corresponding to tag <a>
has a link-related properties, and the one corresponding to <input>
has input-related properties and so on.
Each DOM node belongs to the corresponding built-in class. The root of the hierarchy is EventTarget, that is inherited by Node, and other DOM nodes inherit from it.
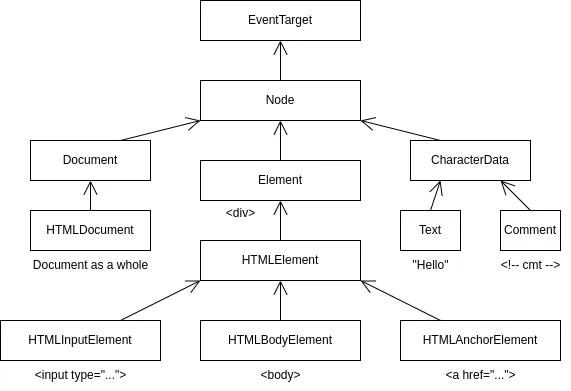
To see the DOM node class name, we can recall that an object ususally has the constructor
property. It references the class constructor, and constructor.name
is its name:
alert(document.body.constructor.name); // HTMLBodyElement
The nodeType
property
The nodeType
property provides one more, old-fashioned
way to get the type
of a DOM node.
It has a numeric value:
elem.nodeType == 1
for element nodes,elem.nodeType == 3
for text nodes,elem.nodeType == 9
for the document object,There are few other values in the specification
In modern scripts, we can use instanceof
and other class-based tests to see the node type, but sometimes nodeType
may be simpler.
Tag: nodeName and tagName
Given a DOM node, we can read its tag name from nodeName
or tagName
properties:
alert(document.body.nodeName); // BODY
alert(document.body.tagName); // BODY
Differences between tagName
and nodeName
:
the
tagName
property exists only forElement
nodes.the
nodeName
is defined for anyNode
:For elements it means the same as
tagName
.For other node types (text, comment, etc...) it has a string with the node type.
innerHTML: the contents
The innerHTML property allows to get the HTML inside the element as a string.
Beware: innerHTML +=
does a full overwrite
We can append HTML like this
elem.innerHTML += "<div>Hello<img src='smile.gif'/> !</div>";
elem.innerHTML += "How goes?";
But we should be very careful about doing it, because what's going on is not an addition, but a full overwrite. Technically, these 2 lines do the same:
elem.innerHTML += "...";
elem.innerHTML = elem.innerHTML + "...";
The old contents is removed
The new
innerHTML
is written instead (a concatenation of the old and the new one).
As the content is
zeroed-outand rewritten from the scratch, all images and other resources will be reloaded.
outerHTML: full HTML of the element
The outerHTML
property contains the full HTML of the element. That's like innerHTML
plus the element itself.
<div id="elem">Hello <b>World</b></div>
<script>
alert(elem.outerHTML);
// element id 'elem'
</script>
Unlike innerHTML
, writing to outerHTML
does not change the element. Instead, it replaces it in the DOM
<div>Hello, world!</div>
<script>
let div = document.querySelector("div");
div.outerHTML = "<p>A new element</p>";
alert(div.outerHTML); // Hello, world!
</script>
What happened in div.outerHTML=...
is:
div
was removed from the document.Another piece of HTML
<p>A new element</p>
was inserted in its place.div
still has its old value. The new HTML wasn't saved to any variable.
nodeValue/data: text node content
The innerHTML
property is only valid for element nodes.
Other node types, such as text nodes, have their counterpart: nodeValue
and data
properties. These two are almost the same for practical use, there are only minor specification differences.
<body>
Hello
<!-- Comment -->
<script>
let text = document.body.firstChild;
alert(text.data); // Hello
let comment = text.nextSibling;
alert(comment.data); // Comment
</script>
</body>
textContent: pure text
The textContent
provides access to the text inside the element: only text, minus all <tags>
<div id="news">
<h1>Headline!</h1>
<p>Martians attack people!</p>
</div>
<script>
// Headline! Martians attack people!
alert(news.textContent);
</script>
Writing to
textContent
is much more useful, because it allows to write text thesafe way
The hidden
property
The hidden
attribute and the DOM property specifies whether the element is visible or not
<div>Both divs below are hidden</div>
<div hidden>With the attribute "hidden"</div>
<div id="elem">JavaScript assigned the property "hidden"</div>
<script>
elem.hidden = true;
</script>
More properties
value
- the value for<input>
,<select>
and<textarea>
(HTMLInputElement
,HTMLSelectElement
...)href
- thehref
for<a href="...">
(HTMLAnchorElement
)id
- the value ofid
attribute, for all elements (HTMLElment
)...and much more...